In Python, both sets and lists are used to store collections of items. However, there are some key differences between the two that are important to understand.
Lists are ordered collections of items, and can contain duplicates. They are mutable, meaning that you can add, remove, or modify items in the list. Lists are created using square brackets, and items are separated by commas.
Sets, on the other hand, are unordered collections of unique items. They cannot contain duplicates, and are mutable. Sets are created using curly braces or the set() function, and items are separated by commas.
One of the main benefits of using sets is that they can be used to perform set operations, such as union, intersection, and difference. Sets are also generally faster than lists for membership testing, since they use a hash table to store their items.
When deciding whether to use a list or a set, it’s important to consider the specific needs of your program. If you need to maintain the order of your items, or if you need to store duplicates, a list may be the better choice. If you need to perform set operations or need to ensure that your items are unique, a set may be the better choice.
Understanding Python Lists
In Python, a list is an ordered and mutable collection of items that can contain items of different data types. Lists can be modified by adding, removing, or modifying items, and can be accessed using indexing and slicing. Python provides built-in functions for working with lists, including len(), min(), max(), and sum(). Lists are a versatile and powerful data structure in Python.
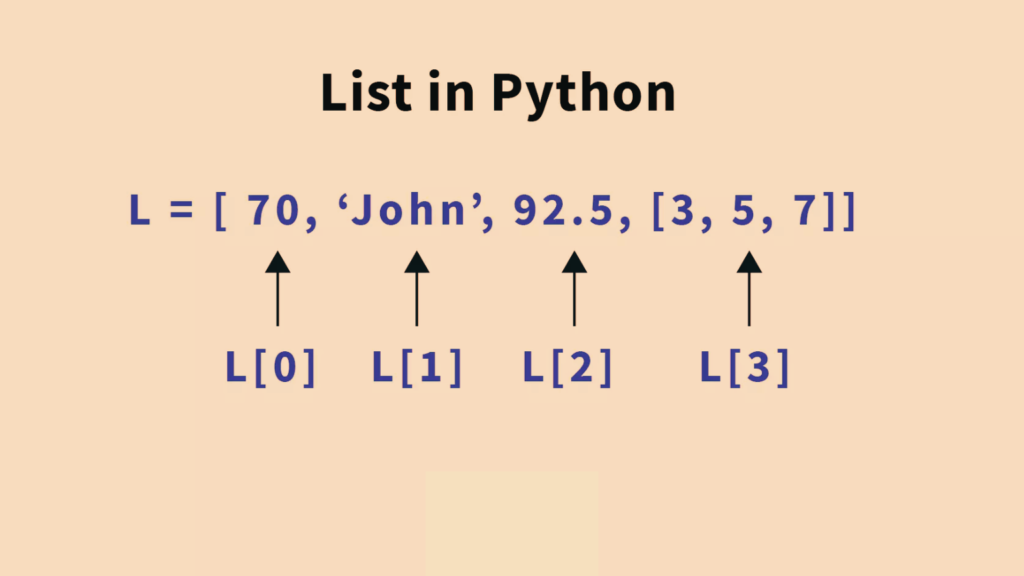
Lists come with various built-in methods for manipulating and accessing the data they hold. Some of these include append() for adding items, remove() for removing items, sort() for sorting the list, and indexing like my_list[0] for accessing the first element.
Power Your Analytics with the Best Business Intelligence Dataset
Exploring Python Sets
In Python, a set is an unordered and mutable collection of unique items. Sets are created using curly braces or the set() function, and items are separated by commas. Sets can be modified by adding or removing items, and can be used to perform set operations such as union, intersection, and difference. Sets are generally faster than lists for membership testing, since they use a hash table to store their items. When deciding whether to use a list or a set, it’s important to consider the specific needs of your program.
Python Set vs List: Key Differences
Uniqueness:
Sets are collections of unique items, while lists can contain duplicate items.
Order:
Sets are unordered, meaning that the items in a set have no specific order, while lists are ordered, meaning that the items in a list are stored in a specific order.
Mutability:
Sets are mutable, meaning that you can add or remove items from a set after it has been created, while lists are also mutable.
Membership testing:
Sets are generally faster than lists for membership testing, since they use a hash table to store their items, while lists must be searched linearly.
When to Use a List vs a Set
You should use a list when you need to maintain the order of the items, and when you need to store duplicate items. Lists are also a good choice when you need to access items by their index, or when you need to modify the items in place. On the other hand, you should use a set when you need to ensure that the items are unique, and when you don’t need to maintain their order.
Sets are also a good choice when you need to perform set operations such as union, intersection, and difference. In general, if you need to perform membership testing frequently, or if you need to remove duplicates from a collection of items, a set is likely to be a better choice than a list. If you need to maintain the order of the items, or if you need to access them by their index, a list is likely to be a better choice.
Accelerate Your Career in AI with Best Machine Learning Courses